
Italy 2024
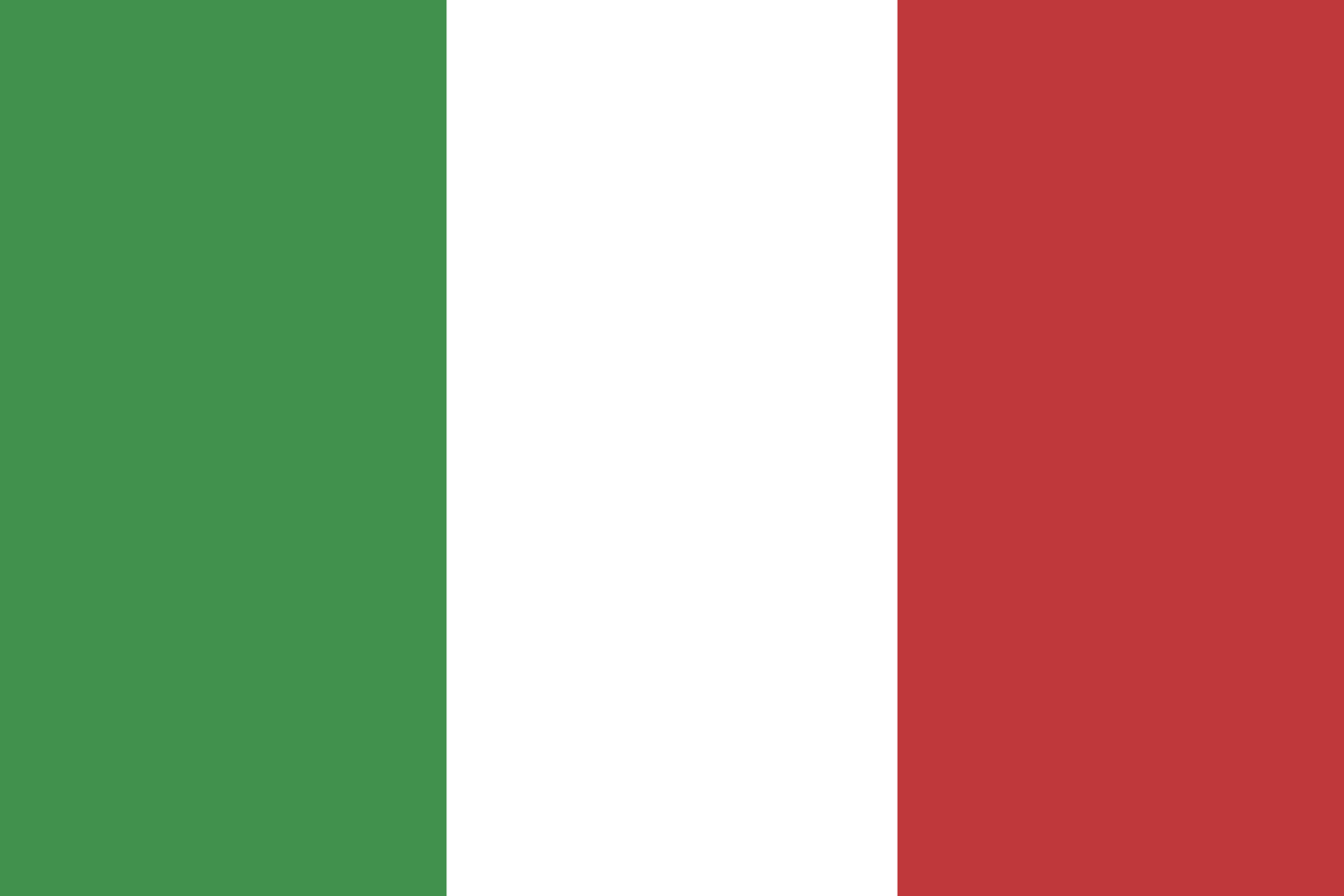
Varese 02.06 - 08.06
Video
Results
Featured
You want to organise a national SGP or even think about the World Finale event? Don’t be shy, it is at the end just welcoming 20 pilots and teams for 10 days! Apply!